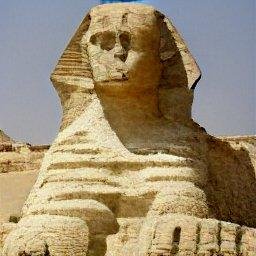
There are so many ways to do this its a bit overwhelming. Here's a simple way to get great looking documentation quickly.
Overview:
- Comment your code with Google docstrings (these are intuitive and lightweight)
- Install
sphinx
(accept all defaults, but ensure to install autodocs and point to your project root) - Install
sphinx_rtd_theme
- Edit
config.py
,index.rst
to include yourmodules cd docs; make html
- BONUS: Check the code into a github repo and point to your repo from readthedocs
That's it, then you'll have GREAT looking docs, and readthedocs.io will host and automatically build your docs with every github commit. Wonderful! Let's get into the details:
1. Comment your code with Google docstrings.
Indentation matters! Here's a simple example using Google docstrings:
def my_func(arg1: int = 5, arg2: str = "hello): """ This line summarizes your function and may have a `link out <http://www.google.com>`_ to another resource. Args: arg1: First parameter arg2: Second parameter Returns (return1, return2) * return1: the first return value
* return2: the second return value
Example Usage First example: >>> print('hello\nworld') .. code-block:: text hello world ... .. image:: images/my_image_in_docs.png Example Usage Secon example: >>> 3 + 5 8 .. image:: images/another_image_in_docs.png
"""
It may also be helpful to use explicit types, like the example above. This resource is useful for that.
2. Install sphinx
The examples use mamba
, which works in conda
environments and tends to run faster. Replace mamba
as desired:
mamba install python3-sphinx
You can accept most of the defaults, but be sure to give the root of your project, and also opt to install the autodocs. The root of your project is the directory where you code lives. So if you have a module called 'my_module' and you want 'docs' to live under 'my_module', then the root will be: ".."
3. Install the sphinx_rtd_theme
This is key, this theme will make your docs gorgeous. While you're at it, install the myst-parser, too, so you can have mark-down; here we use mamba, but conda and pip also work:
mamba install -c conda-forge myst-parser
mamba install sphinx_rtd_theme
After you install these, you'll have to update your config.py
file, which should be in the docs
directory. Add this:
import sphinx_rtd_theme
extensions = [
...
'sphinx_rtd_theme',
]
html_theme = "sphinx_rtd_theme"
Learn more about the style (and see how it should look) here.
4. Edit config.py
, index.rst
to include your "modules"
Follow simple instructions here to set-up your config.py
and index.rst
files so sphinx can find your documented code.
5. cd docs; make html
Build the docs with the commands above, then point your browser at docs/_build/html/index.html to see the results.
6. BONUS: Check the code into a github repo and point readthedocs to your repo
This step is optional, but it can cut back on the size of your github repo and also reduce your bandwidth (github bandwith is currently limited to 1GB/month).
The sbr (click link) repo provides an example of how it all fits together for a pypi module, with module documentation (click link) installed to readthedocs.io
Essential steps:
- Create
docs/requirements.txt
and addmyst-parser
andsphinx_rtd_theme
- Check
docs
into github, e.g.:my_repo/src/my_module/docs
. Be sure to adddocs/config.py
,docs/index.rst
,docs/requirements.txt
, but you may also adddocs/Makefile
. - Add the build targets to your
.gitignore
file; these includedocs/_build
,docs/_templates
- Login to readthedocs.io with your github account and follow the directions to link them up.
- Watch if the build succeeds, otherwise expect to have a few iterations until things are just right. You can click on the steps under the readthedocs.io 'Builds' tab for your project to find out if things are missing from your readthedocs.io deployment based on something you left out of requirements.txt - very helpful! And after you checkin a new requirements.txt be sure to refresh your browser when you visit the website.
Once everything is working, readthedocs will rebuild your docs on every github commit so they're always up to date. Beautiful! Nice job - we covered a lot of ground!
Resources:
- Why use sphinx
Very quick start: https://eikonomega.medium.com/getting-started-with-sphinx-autodoc-part-1-2cebbbca5365
Python built-in types cheat sheet: https://mypy.readthedocs.io/en/stable/cheat_sheet_py3.html#built-in-types
https://sphinxcontrib-napoleon.readthedocs.io/en/latest/example_google.html
https://google.github.io/styleguide/pyguide.html
https://mypy.readthedocs.io/en/stable/cheat_sheet_py3.html
https://sphinx-rtd-theme.readthedocs.io/en/stable/installing.html
https://mypy.readthedocs.io/en/stable/cheat_sheet_py3.html
Also worth a look: https://marketplace.visualstudio.com/items?itemName=njpwerner.autodocstring
- Sphinx quick start