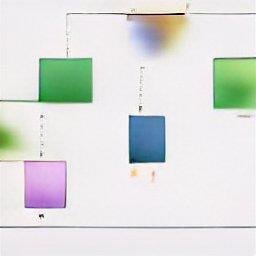
A Python list comprehension is a very convenient syntax for generating simple lists that can replace loops and .append calls to make your code more succinct and readable.
The resources below provide a lot of help for getting started. Here's an example of how a list comprehension can be used to replace a lot of repetitive code with a reusable function:
-extra=(x_train.shape[0] % batch_size) -x_train = x_train[:-extra,:] -#-- -extra=(x_test.shape[0] % batch_size) -x_test = x_test[:-extra,:] -#-- -extra=(x_validation.shape[0] % batch_size) -x_validation = x_validation[:-extra,:] - -extra=(y_train.shape[0] % batch_size) -y_train = y_train[:-extra,:] -#-- -extra=(y_test.shape[0] % batch_size) -y_test = y_test[:-extra,:] -#-- -extra=(y_validation.shape[0] % batch_size) -y_validation = y_validation[:-extra,:]
becomes:
def trim_list_size_to_batch_size_factor(trim_list, batch_size=32): return [ x[:-( x.shape[0] % batch_size ), :] if x.shape[0] % batch_size != 0 else x for x in trim_list ] [x_train, y_train, x_val, y_val, x_test, y_test] = trim_list_size_to_batch_size_factor(trim_list=[x_train, y_train, x_val, y_val, x_test, y_test], batch_size=batch_size)
Resources:
- List comprehensions 101| PyDon't
- CHEAT SHEET: Python list comprehensions 101
- if-else in a list comprehension